
We are going to explain, Custom GraphQL Query Module to fetch the Customer’s Data in Magento 2.x to retrieve basic information of the customer entity as below
- firstname
- lastname
- gender
- dob
- created_at
There are following below steps need to follow.
Customer GraphQL Query Module by using custom module
Here, we are considering as
Namespace / ModuleName = Mage2db / CustomerGraphQl
Step [1] – Create registration.php file under your module [ Namespace / ModuleName ]
File Path=Mage2db/CustomerGraphQl/registration.php
Add below content in this file.
<?php
/**
* @author John
* @copyright Copyright (c) 2022 (https://mage2db.com)
* @package CustomerGraphQl
*/
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Mage2db_CustomerGraphQl',
__DIR__
);
Step [2] – Create module.xml file under your module [ Namespace / ModuleName ]
File Path=Mage2db/CustomerGraphQl/etc/module.xml
Add below content in this file.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Mage2db_CustomerGraphQl" setup_version="1.0.0">
<sequence>
<module name="Magento_GraphQl"/>
<module name="Magento_Backend"/>
<module name="Magento_CustomerGraphQl"/>
</sequence>
</module>
</config>
Briefly Explain registration.php
Our Module is depends on GraphQL and Customer GraphQL Module, we have given dependency on module.xml file.
Step [3] – Every GraphQl Module must contain schema.graphqls file under the etc folder of a module.
File Path=Mage2db/CustomerGraphQl/etc/schema.graphqls
Add below content in this file.
#Custom Module Customer GraphQL
type Query {
customer_details(
id: Int! @doc(description: "Specify The ID of The Customer.")
): CustomerData @resolver( class: "Mage2db\\CustomerGraphQl\\Model\\Resolver\\Customerlist") @doc(description: "Get list of Customer Data for the given customer id.")
}
type CustomerData {
firstname: String
lastname: String
email: String
gender: String
dob: String
city: String
created_at: String
}
id: Int @doc(description: “Id of the Customer”) map to Stored Customers Listing id as Int type
Step [4] – Need to create Customerlist.php file from defined resolver from above schema.
CustomerData @resolver( class: “Mage2db\CustomerGraphQl\Model\Resolver\Customerlist”) @doc(description: “Get list of Customer Data for the given Customer ID.”)
Add below content in this file.
<?php
/**
* @author John
* @email johndusa1021@gmail.com
* @copyright Copyright (c) 2022 (https://mage2db.com)
* @package CustomerGraphQl
*/
namespace Mage2db\CustomerGraphQl\Model\Resolver;
use Magento\Framework\GraphQl\Config\Element\Field;
use Magento\Framework\GraphQl\Exception\GraphQlInputException;
use Magento\Framework\GraphQl\Query\ResolverInterface;
use Magento\Framework\GraphQl\Schema\Type\ResolveInfo;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Framework\GraphQl\Exception\GraphQlNoSuchEntityException;
use Magento\Store\Model\StoreManagerInterface;
class Customerlist implements ResolverInterface
{
protected $_customerSession;
protected $_customerFactory;
protected $_addressFactory;
protected $storeManager;
/**
* @param Field $field
* @param \Magento\Framework\GraphQl\Query\Resolver\ContextInterface $context
* @param ResolveInfo $info
* @param array|null $value
* @param array|null $args
* @return array|\Magento\Framework\GraphQl\Query\Resolver\Value|mixed
* @throws GraphQlInputException
*/
public function __construct(
\Magento\Customer\Model\SessionFactory $customerSession,
\Magento\Customer\Model\CustomerFactory $customerFactory,
\Magento\Customer\Model\AddressFactory $addressFactory,
\Magento\Framework\Url $url,
StoreManagerInterface $storeManager
)
{
$this->_customerSession = $customerSession->create();
$this->_customerFactory = $customerFactory;
$this->_addressFactory = $addressFactory;
$this->urlHelper = $url;
$this->storeManager = $storeManager;
}
public function resolve(
Field $field,
$context,
ResolveInfo $info,
array $value = null,
array $args = null)
{
$currentStoreId = $this->storeManager->getStore()->getId();
$Customer_Collection = $this->_customerFactory->create()->load($args['id']);
return $Customer_Collection->getData();
}
}
As per above script, resolve() method having script which is responsible for getting Customer GraphQl Data.
Step [5] – Finally your Customer GraphQl Query Module has been created
Run the following below commands at your Magento 2 root directory
php bin/magento setup:upgrade
php bin/magento setup:static-content:deploy -f
php bin/magento indexer:reindex
php bin/magento cache:clean
php bin/magento cache:flush
Step [6] – Check your Customer GraphQl Query Module query response by installing chrome extension ChromeiQL or Altair GraphQL addon.
Here we have checked by ChromeiQl
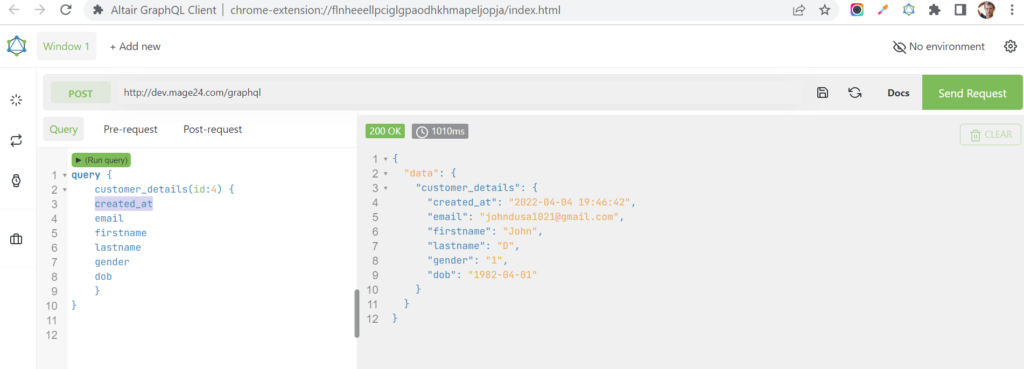