This is a bug in Magento 2.4.3 when using another URL instead of using admin
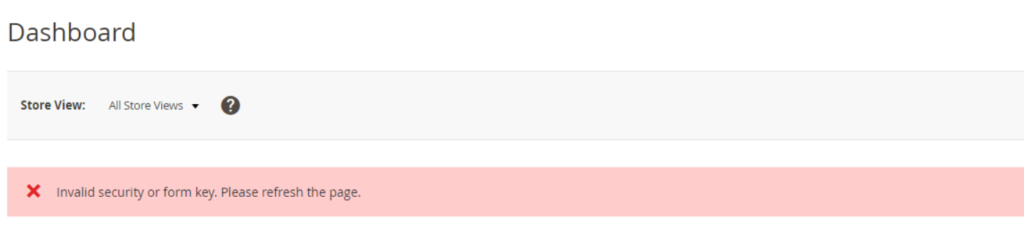
Solution :: Need to change the following below line
\vendor\magento\module-backend\App\Action\Plugin\Authentication.php
Direct modify this file, There is no Magento 2 Standard Code Solution, we are creating custom module to overwrite this file.
The following below steps need to follow.
Step [1] – Create registration.php file under your module [ Namespace / ModuleName ]
File Path=Mage2db/John/registration.php
Add below content in this file.
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Mage2db_John',
__DIR__
);
Step [2] – Create module.xml file under your module [ Namespace / ModuleName ]
File Path=Mage2db/John/etc/module.xml
Add below content in this file.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Mage2db_John" setup_version="1.0.0" />
</config>
Step [3] – Create composer.json file under your module [ Namespace / ModuleName ]
File Path=Mage2db/John/composer.json
Add below content in this file.
{
"name": "Mage2db/John",
"type": "magento2-module",
"description": "This Module is a bug fix for the error message in the dashboard after login into the Magento 2.4.3 backend",
"minimum-stability": "dev",
"require": {
"php": ">=7.3.0"
},
"autoload": {
"files": [
"registration.php"
],
"psr-4": {
"Mage2db\\John\\": ""
}
}
}
Step [4] – di preference for overriding the Authentication.php with our AuthenticationOverride.php
File Path=Mage2db/John/etc/di.xml
Add below content in this file.
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<preference for="Magento\Backend\App\Action\Plugin\Authentication" type="Mage2db\John\App\Action\Plugin\AuthenticationOverride" />
</config>
Step [5] – Finally, replacement of method redirectIfNeededAfterLogin
of below core file path
\vendor\magento\module-backend\App\Action\Plugin\Authentication.php
with our custom code
Add AuthenticationOverride.php in the below path & code
File Path as below
Mage2db/John /App/Action/Plugin/AuthenticationOverride.php
<?php
namespace Mage2db\John\App\Action\Plugin;
use Magento\Framework\Exception\AuthenticationException;
/**
* @SuppressWarnings(PHPMD.CouplingBetweenObjects)
*/
class AuthenticationOverride
{
/**
* @var \Magento\Backend\Model\Auth
*/
protected $_auth;
/**
* @var string[]
*/
protected $_openActions = [
'forgotpassword',
'resetpassword',
'resetpasswordpost',
'logout',
'refresh', // captcha refresh
];
/**
* @var \Magento\Backend\Model\UrlInterface
*/
protected $_url;
/**
* @var \Magento\Framework\App\ResponseInterface
*/
protected $_response;
/**
* @var \Magento\Framework\App\ActionFlag
*/
protected $_actionFlag;
/**
* @var \Magento\Framework\Message\ManagerInterface
*/
protected $messageManager;
/**
* @var \Magento\Backend\Model\UrlInterface
*/
protected $backendUrl;
/**
* @var \Magento\Backend\App\BackendAppList
*/
protected $backendAppList;
/**
* @var \Magento\Framework\Controller\Result\RedirectFactory
*/
protected $resultRedirectFactory;
/**
* @var \Magento\Framework\Data\Form\FormKey\Validator
*/
protected $formKeyValidator;
/**
* @param \Magento\Backend\Model\Auth $auth
* @param \Magento\Backend\Model\UrlInterface $url
* @param \Magento\Framework\App\ResponseInterface $response
* @param \Magento\Framework\App\ActionFlag $actionFlag
* @param \Magento\Framework\Message\ManagerInterface $messageManager
* @param \Magento\Backend\Model\UrlInterface $backendUrl
* @param \Magento\Framework\Controller\Result\RedirectFactory $resultRedirectFactory
* @param \Magento\Backend\App\BackendAppList $backendAppList
* @param \Magento\Framework\Data\Form\FormKey\Validator $formKeyValidator
*/
public function __construct(
\Magento\Backend\Model\Auth $auth,
\Magento\Backend\Model\UrlInterface $url,
\Magento\Framework\App\ResponseInterface $response,
\Magento\Framework\App\ActionFlag $actionFlag,
\Magento\Framework\Message\ManagerInterface $messageManager,
\Magento\Backend\Model\UrlInterface $backendUrl,
\Magento\Framework\Controller\Result\RedirectFactory $resultRedirectFactory,
\Magento\Backend\App\BackendAppList $backendAppList,
\Magento\Framework\Data\Form\FormKey\Validator $formKeyValidator
) {
$this->_auth = $auth;
$this->_url = $url;
$this->_response = $response;
$this->_actionFlag = $actionFlag;
$this->messageManager = $messageManager;
$this->backendUrl = $backendUrl;
$this->resultRedirectFactory = $resultRedirectFactory;
$this->backendAppList = $backendAppList;
$this->formKeyValidator = $formKeyValidator;
}
/**
* @param \Magento\Backend\App\AbstractAction $subject
* @param \Closure $proceed
* @param \Magento\Framework\App\RequestInterface $request
*
* @return mixed
* @SuppressWarnings(PHPMD.UnusedFormalParameter)
*/
public function aroundDispatch(
\Magento\Backend\App\AbstractAction $subject,
\Closure $proceed,
\Magento\Framework\App\RequestInterface $request
) {
$requestedActionName = $request->getActionName();
if (in_array($requestedActionName, $this->_openActions)) {
$request->setDispatched(true);
} else {
if ($this->_auth->getUser()) {
$this->_auth->getUser()->reload();
}
if (!$this->_auth->isLoggedIn()) {
$this->_processNotLoggedInUser($request);
} else {
$this->_auth->getAuthStorage()->prolong();
$backendApp = null;
if ($request->getParam('app')) {
$backendApp = $this->backendAppList->getCurrentApp();
}
if ($backendApp) {
$resultRedirect = $this->resultRedirectFactory->create();
$baseUrl = \Magento\Framework\App\Request\Http::getUrlNoScript($this->backendUrl->getBaseUrl());
$baseUrl = $baseUrl . $backendApp->getStartupPage();
return $resultRedirect->setUrl($baseUrl);
}
}
}
$this->_auth->getAuthStorage()->refreshAcl();
return $proceed($request);
}
/**
* Process not logged in user data
*
* @param \Magento\Framework\App\RequestInterface $request
* @return void
*/
protected function _processNotLoggedInUser(\Magento\Framework\App\RequestInterface $request)
{
$isRedirectNeeded = false;
if ($request->getPost('login')) {
if ($this->formKeyValidator->validate($request)) {
if ($this->_performLogin($request)) {
$isRedirectNeeded = $this->_redirectIfNeededAfterLogin($request);
}
} else {
$this->_actionFlag->set('', \Magento\Framework\App\ActionInterface::FLAG_NO_DISPATCH, true);
$this->_response->setRedirect($this->_url->getCurrentUrl());
$this->messageManager->addErrorMessage(__('Invalid Form Key. Please refresh the page.'));
$isRedirectNeeded = true;
}
}
if (!$isRedirectNeeded && !$request->isForwarded()) {
if ($request->getParam('isIframe')) {
$request->setForwarded(true)
->setRouteName('adminhtml')
->setControllerName('auth')
->setActionName('deniedIframe')
->setDispatched(false);
} elseif ($request->getParam('isAjax')) {
$request->setForwarded(true)
->setRouteName('adminhtml')
->setControllerName('auth')
->setActionName('deniedJson')
->setDispatched(false);
} else {
$request->setForwarded(true)
->setRouteName('adminhtml')
->setControllerName('auth')
->setActionName('login')
->setDispatched(false);
}
}
}
/**
* Performs login, if user submitted login form
*
* @param \Magento\Framework\App\RequestInterface $request
* @return bool
*/
protected function _performLogin(\Magento\Framework\App\RequestInterface $request)
{
$outputValue = true;
$postLogin = $request->getPost('login');
$username = isset($postLogin['username']) ? $postLogin['username'] : '';
$password = isset($postLogin['password']) ? $postLogin['password'] : '';
$request->setPostValue('login', null);
try {
$this->_auth->login($username, $password);
} catch (AuthenticationException $e) {
if (!$request->getParam('messageSent')) {
$this->messageManager->addErrorMessage($e->getMessage());
$request->setParam('messageSent', true);
$outputValue = false;
}
}
return $outputValue;
}
/**
* Checks, whether Magento requires redirection after successful admin login, and redirects user, if needed
*
* @param \Magento\Framework\App\RequestInterface $request
* @return bool
*/
protected function _redirectIfNeededAfterLogin(\Magento\Framework\App\RequestInterface $request)
{
$requestUri = null;
// Checks, whether secret key is required for admin access or request uri is explicitly set
if ($this->_url->useSecretKey()) {
$requestParts = strpos(trim($request->getRequestUri(),'/'), $request->getFrontName()) === 0 ?
explode('/', trim($request->getRequestUri(), '/'), 4) :
explode('/', trim($request->getRequestUri(), '/'), 3);
if (($key = array_search($request->getFrontName(), $requestParts)) !== false) {
unset($requestParts[$key]);
}
$requestParams = $request->getParams();
unset($requestParams['key'], $requestParams['form_key']);
$requestUri = $this->_url->getUrl(implode('/', $requestParts), $requestParams);
} elseif ($request) {
$requestUri = $request->getRequestUri();
}
if (!$requestUri) {
return false;
}
$this->_response->setRedirect($requestUri);
$this->_actionFlag->set('', \Magento\Framework\App\ActionInterface::FLAG_NO_DISPATCH, true);
return true;
}
}
Finally, run below CLI command & issue will be resolved once admin login.
php bin/magento cache:clean
php bin/magento cache:flush
php bin/magento indexer:reindex
php bin/magento setup:upgrade
php bin/magento setup:static-content:deploy -f
Other important Magento 2.4.x issue as below
Magento 2.3 To Magento 2.4.5 Comptibility For PHP, MYSQL, Composer, Apache etc
Magento 2.4.3-p1 Installation Steps
Magento 2.4.3 Installation Steps
Magento 2.4 Two Factor Authentication
Magento 2.2, Magento 2.3, Magento2.4 Installation Issue on Windows 10, XAMPP
Magento 2 Installation at 51% Error: (Wrong file in Gd2.php:64) Module ‘Magento_Theme’