
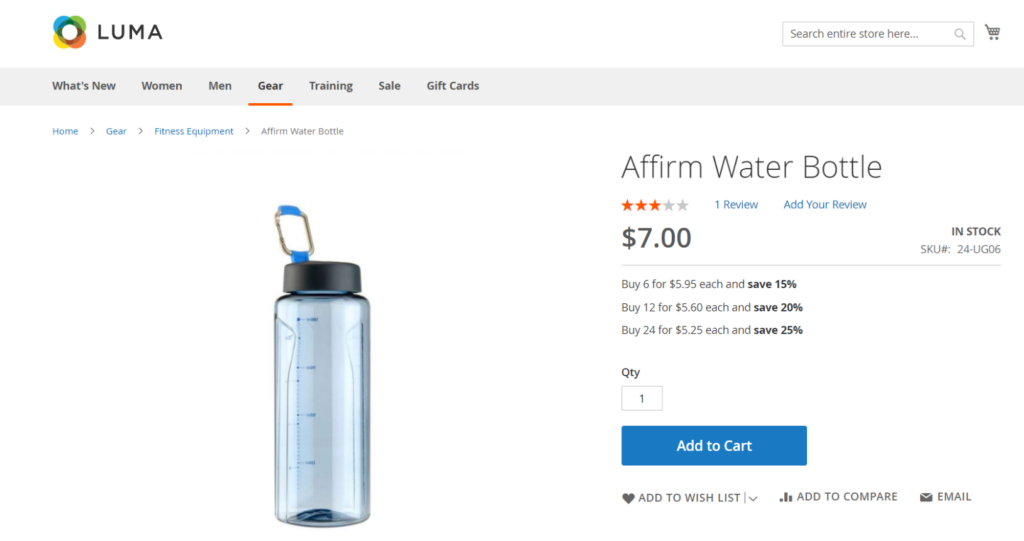
There are following below steps to create custom module to add or update tier price in Magento2
Here, we are considering as
Namespace / ModuleName = Mage2db/John
Step [1.1] – Create registration.php file under your module [ Namespace / ModuleName ]
File Path=Mage2db/John/registration.php
Add below content in this file.
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Mage2db_John',
__DIR__
);
Step [1.2] – Create module.xml file under your module [ Namespace / ModuleName ]
File Path=Mage2db/John/etc/module.xml
Add below content in this file.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Mage2db_John" setup_version="1.0.0" />
</config>
Step [2] – Create Index.php file under your module Controller Index directory. Add below content in this file.
File Path=Mage2db/John/Controller/Index/Index.php
Here, we are using below core class to add tier or customer group price
Magento\Catalog\Api\Data\ProductTierPriceInterfaceFactory;
Magento\Catalog\Api\ScopedProductTierPriceManagementInterface;
<?php
namespace Mage2db\John\Controller\Index;
use Magento\Backend\App\Action\Context;
use \Magento\Framework\Controller\ResultFactory;
use Magento\Catalog\Api\Data\ProductTierPriceInterfaceFactory;
use Magento\Catalog\Api\ScopedProductTierPriceManagementInterface;
class Index extends \Magento\Framework\App\Action\Action
{
/**
* @var \Magento\Framework\App\Cache\TypeListInterface
*/
protected $_cacheTypeList;
/**
* @var \Magento\Framework\App\Cache\StateInterface
*/
protected $_cacheState;
/**
* @var \Magento\Framework\App\Cache\Frontend\Pool
*/
protected $_cacheFrontendPool;
/**
* @var \Magento\Framework\View\Result\PageFactory
*/
protected $resultPageFactory;
/**
* @var ScopedProductTierPriceManagementInterface
*/
private $tierPrice;
/**
* @var ProductTierPriceInterfaceFactory
*/
private $productTierPriceFactory;
/**
* @param Action\Context $context
* @param \Magento\Framework\App\Cache\TypeListInterface $cacheTypeList
* @param \Magento\Framework\App\Cache\StateInterface $cacheState
* @param \Magento\Framework\App\Cache\Frontend\Pool $cacheFrontendPool
* @param \Magento\Framework\View\Result\PageFactory $resultPageFactory
*/
public function __construct(
\Magento\Framework\App\Action\Context $context,
\Magento\Framework\App\Cache\TypeListInterface $cacheTypeList,
\Magento\Framework\App\Cache\StateInterface $cacheState,
\Magento\Framework\App\Cache\Frontend\Pool $cacheFrontendPool,
\Magento\Framework\Message\ManagerInterface $messageManager,
\Magento\Framework\View\Result\PageFactory $resultPageFactory,
ScopedProductTierPriceManagementInterface $tierPrice,
ProductTierPriceInterfaceFactory $productTierPriceFactory
) {
parent::__construct($context);
$this->_cacheTypeList = $cacheTypeList;
$this->_cacheState = $cacheState;
$this->_cacheFrontendPool = $cacheFrontendPool;
$this->resultPageFactory = $resultPageFactory;
$this->_messageManager = $messageManager;
$this->tierPrice = $tierPrice;
$this->productTierPriceFactory = $productTierPriceFactory;
}
/**
* Flush cache storage
*
*/
public function execute()
{
$qty = 2.00;//Quantity must be float value
$price = 300.00;//Price must be float value
$customerGroupId = 4; //CustomerGroup ID
$sku = 'product1021'; //put sku,to add tier price
/*As per your need all above values create dynamically */
try {
$tierPriceData = $this->productTierPriceFactory->create();
$tierPriceData->setCustomerGroupId($customerGroupId)->setQty($qty)->setValue($price);
$tierPrice = $this->tierPrice->add($sku, $tierPriceData);
} catch (NoSuchEntityException $exception) {
throw new NoSuchEntityException(__($exception->getMessage()));
}
echo"Tier Price or Customer Group Price has been updated for SKU=$sku";
exit();
$this->resultPage = $this->resultPageFactory->create();
return $this->resultPage;
}
}
Step [3] – Create routes.xml file under your module etc directory. Add below content in this file.
File Path=Mage2db\John\etc\frontend\routes.xml
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../../../../../../lib/internal/Magento/Framework/App/etc/routes.xsd">
<router id="standard">
<route id="john" frontName="john">
<module name="Mage2db_John" />
</route>
</router>
</config>
Step [3] – Run below URL
https://mage2db.com/john/index/index
Change https://mage2db.com to your Base URL.
Finally Tier Price / Customer Group Price, custom module has been created.
How To Create Events & Observer Using Custom Module in Magento / Adobe Commerce 2.x
How To Create Preference Using Custom Module in Magento / Adobe Commerce 2.x
How To Create Plugin Using Custom Module in Magento / Adobe Commerce 2.x
How To Get Base URL in Magento 2.x / Adobe Commerce 2.x
How To Create Custom Module in Magento 2.x / Adobe Commerce 2.x
How To Add Custom Block on Cart Page in Magento 2.x / Adobe Commerce 2.x
How To Create a Custom Log File in Magento 2.x / Adobe Commerce 2.x
How To Create Custom Controller in Magento 2.x / Adobe Commerce 2.x
How To Create a Custom Console Command in Magento 2.x / Adobe Commerce 2.x
How To Get all Customers Data in Magento 2.x / Adobe Commerce 2.x
How To Set Tier Price With Percentage Value Programmatically in Magento 2.x / Adobe Commerce 2.x
How To Add Tier Price Programmatically in Magento 2.x / Adobe Commerce 2.x
Magento 2 All Database Tables [500 & more Tables]
How To Set Multi Shipping Settings In Magento 2
How To Set Origin Shipping in Magento 2
Difference Between Offline Shipping Method and Online Shipping Method
Magento 2 Online Customers Options
How To Apply Customer Group Price of Products in Magento 2
How To Add Customer Groups Dropdown in Magento 2 Admin Form and Grid By UI Component
How To Get all Customers Data in Magento 2
How To Create Customer Order in Magento 2 Admin Panel
Magento 2 Login As Customer Not Enabled
How To Configure Customer Account Sharing Options in Magento 2
Magento 2 Redirect To Customer Dashboard After Login
Which Magento 2 database table store customer shipping and billing address
How To Remove Sales Order Data & Customer Data in Magento 2
Which Magento 2 database table store customer’s Email Data
Which Magento 2 Database Table Store Customer Newsletter Data
Which Magento 2 database table store customer’s shipping and billing address
How To Remove Sales Order Data & Customer Data in Magento 2
Which Magento 2 Database Tables Store Customer Rating
Which Magento 2 Database Tables Store Customer Wishlist Products
Magento 2 Increase Customer Session Time